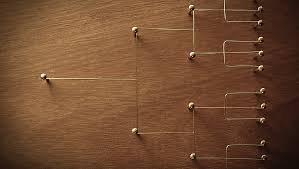
Grouping data in C can be done using C structures. These c structures can be grouped as an array of structs if need be. The advantage of using structures in c allows the programmer to better pass data between functions, easier access to specific fields within that structure, can use typedef’s to better represent that data. Structures are used to create linked lists, binary trees, among other data structures. The question is, how do we create and use these structures?
I hope to outline the basics of a C struct and its many uses in this article.
What Does a ‘C Structure’ Look Like And How Do We Use It?
A structure in C is nothing special really, once you get the syntax down they are a piece of cake, for example:
struct data {
int number;
char *name;
double id;
};
This is a structure named ‘data’. To create an instance of one there are a few options:
struct data data_one; // This creates a single data structure called data_one
OR in our definition we can do:
struct data {
int number;
char *name;
double id;
} data_one;
This defines and creates 1 structure of data called data_one. Now that we have a single structure called data_one, how can we access the variables within it? Because we are referencing the structure directly, rather than using a pointer to it, we use the . . For example:
data_one.number = 5;
data_one.name = &some_string_address;
data_one.id = 12;
Now our structure contains those values, the beauty of this is we can just pass the structure around, rather than passing all three variables around at once. This is somewhat cumbersome to create structures this way, instead we could create a type using typedef.
Creating a ‘C’ Struct As a Type
Okay, lets say we are planning to use our structure “data” a lot, and we would be creating many of them. We can create a type to easily create new instances of this structure in our code.
struct data {
int number;
char *name;
double id;
};
typedef struct data data_t;
We now have a data type called data_t that is actually our data structure. Cool! So what does that mean? This means that we have a data type just like an int, or a float, its just our data type happens to be a structure containing some of those types. This makes it quite easy to make new instances of our structure.
data_t eriks_data;
eriks_data.number = 5;
eriks_data.id = 12;
// Or create a data structure on the fly for me to point to.
data_t *eriks_data_struct_ptr = (data_t *)malloc(sizeof(data_t));
if(eriks_data_struct_ptr)
eriks_data_struct_ptr -> number = 5;
The above example shows how we can take advantage of the typedef’d data_t but also how to create an instance of our structure on the fly using malloc and pointers. In the pointer example, we reference the member number differently using the -> symbol indicating we are pointing rather than referencing directly.
Create Array Of ‘C’ Structures
So now we have the data type data_t for use just like any other data type. Lets say you know you need twenty (20) of them. Well just like any other array, we can simply do:
data_t eriks_data[20]; // This will create twenty instances of our structure.
Or, if we had not created our type using typedef we can just do:
struct data eriks_data[20];
Its really that easy. You can think of our type definition as any other type.
Comments