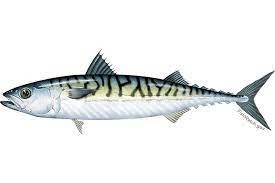
I often find myself copying and pasting C macros from one program to another. Evidently I end up using these macros more often than I thought. So I thought I would share these useful macros with you. I will write about C macros later, C debugging macros and a guide to using C macros in your code. I find them quite useful, but how do macros affect the final product?
A macro (if not C99) is actually an inline expansion of your code. This means that the compiler will insert this code block directly into every spot you refer to the macro, rather than referencing the code block via a memory location. This can save pointer referencing and in some cases your code will be more efficient. You cannot, however, ensure this is the case the compiler may make decisions that it believes will be more efficient. None the less here is a list of some useful macros.
A Macro To Detect File Presence
Rather than attempting to open a file, you can use this macro:
#define exists(filename) (!access(filename, F_OK))
In your code you can then do something like:
if(exists("data.txt"))
.. open file ...
else
Get The Size of An Array of Arbitrary Type
There are some instances where you don’t actually know the size of your array. This handy macro will tell you the size of your array, i.e. how many elements are in it:
#define ARRAY_SIZE(x) (sizeof(x) / sizeof((x)[0]))
This example utilizes the sizeof operator. You can use this macro as such:
int data[50];
fprintf(stdout, "Array size: %u\n",ARRAY_SIZE(data));
Min and Max
The always handy minimum and maximum macros will return the smaller or the larger of two items. These items can be of an arbitrary type, as long as they are the same type.
#define min(x,y) ({ \
typeof(x) _x = (x); \
typeof(y) _y = (y); \
(void) (&_x == &_y); \
_x < _y ? _x : _y; })
#define max(x,y) ({ \
typeof(x) _x = (x); \
typeof(y) _y = (y); \
(void) (&_x == &_y); \
_x > _y ? _x : _y; })
To use these macros, is much like any other:
int main()
{
int a,b;
a=5;
b=12;
fprintf(stdout, "%u\n", min(a,b));
return 0;
}
$ ./min
5
Voila, here are a few of the macros I find myself commonly using.
Comments